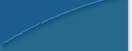
|
//-----------------------------------------------------------------------------
// Greenhouse.vpl, created 2000-05-16 09:01
// This program solves the following job:
//
// Gardener Green has a greenhouse in which he wants to control the temperature.
// For that purpose he has bought a couple of temperature sensors and some
// actuators. One of the temperature sensors will be adjusted to give a
// signal when the temperature falls below a given temperature, and the
// other sensor will give a signal when the temperature rises above a given
// temperature. Using inputs from these sensores he wants to turn on a
// heating unit and turn on the ventilation duct.
// If the system does not succeed in bringing the temperature within the
// given limits, the gardener want's to have a SMS message sent to his
// mobile telephone. This message will notify him about the status of the
// greenhouse.
//-----------------------------------------------------------------------------
INCLUDE rtcu.inc
VAR_INPUT
Sensor_L : BOOL; | Sensorinput for low temperature
Sensor_H : BOOL; | Sensorinput for high temperature
Alarm_time : INT; | Time, 0..32767 minutes, time before SMS message is sent
phone_number : SRING; | Telephone number to send SMS message to
END_VAR;
VAR_OUTPUT
Out_Heater : BOOL; | Output to activate heater
Out_Ventilation : BOOL; | Output to activate ventilation
online : BOOL; | Is unit connected to a GSM basestation
END_VAR;
VAR
timer : TON; // ON-delay timer is declared.
// A On-delay timer goes active when 'trig' has
// been active for 'pt' seconds.
send_alarm : R_TRIG; // detects leading edge on alarm
END_VAR;
PROGRAM greenhouse;
// Initialization code:
// Set the timer
// (Convert to milliseconds)
timer(pt := Alarm_time*60*1000);
// turn on GSM-module
gsmPower(power := ON);
BEGIN
timer();
// Update status for connected to GSM base station
online := gsmConnected();
// If the temperature is to high then activate ventilation:
IF Sensor_H THEN
Out_Ventilation:= ON;
ELSE
Out_Ventilation:= OFF;
END_IF;
// If the temperature is to low then activate the heater:
IF Sensor_L THEN
Out_Heater:= ON;
ELSE
Out_Heater:= OFF;
END_IF;
// Start timer on the leading edge of the sensor-inputs:
timer(trig:= Sensor_L OR Sensor_H);
// Detect leading edge on alarm:
send_alarm(trig:=timer.q);
IF send_alarm.q THEN
IF Sensor_L THEN
// send SMS for temperature too low
gsmSendSMS(phonenumber:=phone_number, message:="Temperature too low");
ELSIF Sensor_H THEN
// send SMS for temperature too high
gsmSendSMS(phonenumber:=phone_number, message:="Temperature too high");
END_IF;
END_IF;
END;
END_PROGRAM;
|
|