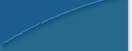
|
//-----------------------------------------------------------------------------
// Datalogger testprogram
//-----------------------------------------------------------------------------
INCLUDE rtcu.inc
VAR
LogWriter : LogWrite; // FB used for writing log entries
LogReader : LogRead; // FB used for reading entry at current read position
END_VAR;
//---------------------------------------------------------------------------
// Function that will show a text and a number
//---------------------------------------------------------------------------
FUNCTION ShowNum;
VAR_INPUT
msg : STRING;
num : DINT;
END_VAR
DebugMsg(message:=strConcat(str1:=msg, str2:=strFormat(format:="\4", v4:=num)));
END_FUNCTION;
//---------------------------------------------------------------------------
// Mainprogram
//---------------------------------------------------------------------------
PROGRAM test;
// Test if the Datalogger is initialized
IF NOT LogIsInitialized(key:=4712) THEN
// Initialize datalogger system, 2 values per record, 10 records in total
LogInitialize(key:=4712, numlogvalues:=2, numlogrecords:=10);
DebugMsg(message:="Log initialized");
ELSE
DebugMsg(message:="Log was already initialized");
END_IF;
// Check how many records we have room for in the datalogger
// Should be 2
ShowNum(msg:="(a) LogMaxNumOfRecords() = ", num:=LogMaxNumOfRecords());
// Check how many values in each record
// Should be 2
ShowNum(msg:="(b) LogValuesPerRecord() = ", num:=LogValuesPerRecord());
// Make an entry in the datalogger
LogWriter(tag:=1, value[1]:=10, value[2]:=20);
// And another
LogWriter(tag:=2, value[1]:=11, value[2]:=21);
// Check how many records present in the datalogger
// Should be 2
ShowNum(msg:="(c) LogNumOfRecords() = ", num:=LogNumOfRecords());
// Set read position to the oldest (first) record
LogFirst();
// Fetch data from read position
LogReader(); // LogReader.tag is 1, value[1] is 10, value[2] is 20
// Advance read position (forward in time)
LogNext();
LogReader(); // LogReader.tag is 2, value[1] is 11, value[2] is 21
// LogReader.tag should be 2
ShowNum(msg:="(d) LogReader.tag = ", num:=LogReader.tag);
// Advance read position (backward in time)
// We are now back to the first record
LogPrev();
LogReader(); // LogReader.tag is 1, value[1] is 10, value[2] is 20
// LogReader.tag should be 1
ShowNum(msg:="(e) LogReader.tag = ", num:=LogReader.tag);
// Modify the current record
LogReWriteTag(tag:=123); // This will change the tagvalue of the current record to 123
// Now read record
LogReader(); // LogReader.tag is now 123, value[1] is 10, value[2] is 20
// LogReader.tag should be 123
ShowNum(msg:="(f) LogReader.tag = ", num:=LogReader.tag);
ShowNum(msg:="(f) LogReader.year = ", num:=LogReader.year);
ShowNum(msg:="(f) LogReader.month = ", num:=LogReader.month);
ShowNum(msg:="(f) LogReader.day = ", num:=LogReader.day);
ShowNum(msg:="(f) LogReader.hour = ", num:=LogReader.hour);
ShowNum(msg:="(f) LogReader.minute = ",num:=LogReader.minute);
ShowNum(msg:="(f) LogReader.second = ",num:=LogReader.second);
BEGIN
END;
END_PROGRAM;
|
|