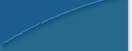
|
//-----------------------------------------------------------------------------
// GPS Mobile.vpl, created 2002-05-30 09:30
//
// Small application that shows how to obtain GPS positions from the external
// GPS receiver on the RTCU product. The program will show in the debug window
// (typically in the simulator) the current distance and bearing to Logic IO in
// Denmark. If an SMS text message is sent to the RTCU unit, it will respond
// back to the senders phone with the current distance/bearing to Logic IO
//
// Please note that the LED1/2 indicator will be:
// green if connected to GSM net but NO valid GPS position
// red if GPS position valid but NOT connected to GSM net
// orange (green and red) if connected to GSM net and valid GPS position
//-----------------------------------------------------------------------------
INCLUDE rtcu.inc
VAR_OUTPUT
gsmConnect : BOOL; | Indicates that the GSM is connected to a basestation (Green)
gpsValid : BOOL; | Indicates that a position fix has been obtained (Red)
END_VAR;
PROGRAM GPS_Example;
VAR
sms : gsmIncomingSMS;
gps : gpsFix;
gc : gpsDistanceX;
str : string;
awaitFix : bool;
END_VAR;
// Turn on power to the GPS receiver
gpsPower(power:=true);
// Turn on power to the GSM module
gsmPower(power:=true);
BEGIN
sms();
// Update GPS data
gps();
// If we got a valid fix from the GPS
if gps.mode > 1 then
// Calculate distance and bearing to Logic IO in Denmark
gc(latitude1:=55513078, longitude1:=9510530, latitude2:=gps.latitude, longitude2:=gps.longitude);
// Build string with information
str:=strFormat(format:="Distance to Logic IO=\4.\3 KM, bearing=\2 deg", v4:=gc.distance/1000, v3:=int(gc.distance mod 1000), v2:=gc.bearing);
end_if;
// If we receive a SMS message, we want the next GPS position that is valid
if sms.status>0 then
awaitFix:=true;
end_if;
// If we are waiting for next valid GPS position, and GPS position is valid,
if awaitFix and gps.mode > 1 then
awaitFix:=false;
// Send an SMS with the position
gsmSendSMS(phonenumber:=sms.phonenumber, message:=str);
end_if;
// Indicate on LED (green part) if we are connected to a GSM basestation
gsmConnect:=gsmConnected();
// Indicate on LED (red part) if valid GPS position
gpsValid := gps.mode > 1;
END;
END_PROGRAM;
|
|