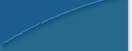
|
//--------------------------------------------------------------------------
// This program extends the basic Greenhouse control program in that
// a connected lamp in the greenhouse can be turned on/off in several
// different ways:
// -Sending an SMS message to the unit. By sending the message
// "ON" the lamp will turn on. Sending the message "OFF" will turn
// off the lamp.
//
// -Calling the unit and control it by voice/keypress interaction
// (Voice Response System).
// When the unit answers the call it will greet the caller with
// the message:
// "Press 1 to activate lamp, press 0 to deactivate the lamp"
// The caller can then press '0' or '1'. Any other key wil result
// in the message: "Illegal keypress"
// When a legal command is received the unit says:
// "Have a nice day".
//
// The conneced lamp must be initially turned on when the unit powers up.
//
// In addition to the lamp there is also a door-contact used for detecting
// when the door is opened. If this happens and the feature is enabled the
// unit will call the gardener and say "Burgler in the greenhouse"
// This alarmfeature can be enabled/disabled with a Keyswitch that is mounted
// at the entrance to the greenhouse.
//--------------------------------------------------------------------------
INCLUDE rtcu.inc
VAR_INPUT
Sensor_L : BOOL; | Sensorinput for low temperature
Sensor_H : BOOL; | Sensorinput for high temperature
Alarm_time : INT; | time, 1..32767 minutter, before SMS message is sent
Doorcontact : BOOL R_EDGE; | Doorcontact that will ignite the burglar-alarm
Alarm_keyswitch: BOOL; | Is the burglar-alarm activated?
PhoneNo : STRING; | Phonenumber to call when burglar-alarm occurs
END_VAR;
VAR_OUTPUT
Out_Heater : BOOL; | Output to activate heater
Out_Ventilation: BOOL; | Output to activate ventilation
Lamp : BOOL:=ON; | Lamp (initially ON)
Connected : BOOL; | The unit is connected to the GSM-network.
OffHook : BOOL; | "off-hook" condition.
END_VAR;
VAR
timer : TON; // ON-delay timer is declared.
// A On-delay timer goes active when 'trig' has
// been active for 'pt' seconds.
send_alarm : R_TRIG; // detects leading edge on alarm
state : INT := 1; // State for Voice call
sms : gsmIncomingSMS;
voicecall : gsmIncomingCall;
END_VAR;
//---------------------------------------------------------------------------
// Control the temperature in the greenhouse, and send SMS message
// if temperature is outside limits for a specified period
//---------------------------------------------------------------------------
FUNCTION TemperatureControl;
// if sensor for high temp. is activated we turn on the ventilation:
Out_Ventilation := Sensor_H;
// if sensor for low temp. is activated we turn on the heater:
Out_Heater := Sensor_L;
// Start timer on the leading edge of the sensor-inputs:
timer(trig:= Sensor_L OR Sensor_H);
// Detect leading edge on alarm:
send_alarm(trig:=timer.q);
IF send_alarm.q THEN
IF Sensor_L THEN
// send sms for temperature too low
gsmSendSMS(phonenumber:=PhoneNo, message:="Temperature too low");
ELSIF Sensor_H THEN
// send sms for temperature too high
gsmSendSMS(phonenumber:=PhoneNo, message:="Temperature too high");
END_IF;
END_IF;
END_FUNCTION;
//---------------------------------------------------------------------------
// Control the lamp in the greenhouse using a SMS message
//---------------------------------------------------------------------------
FUNCTION SMSLamp;
// Check if we have received a SMS message:
IF sms.status > 0 THEN
// SMS message received
// If it's a "ON" message
// (Note: strCompare doesn't check the case by default)
IF strCompare(str1:=sms.message, str2:="ON")=0 THEN
lamp:=ON;
// Else if it's a "OFF" message
ELSIF strCompare(str1:=sms.message, str2:="OFF")=0 THEN
lamp:=OFF;
END_IF;
END_IF;
END_FUNCTION;
//---------------------------------------------------------------------------
// Control the lamp in the greenhouse using a simple voice-response system
//---------------------------------------------------------------------------
FUNCTION VoiceLamp;
VAR
key : INT; // key selected in voice-menu
END_VAR;
// Voice/response:
CASE state OF
1: // waiting for call:
IF voicecall.status > 0 THEN
gsmAnswer();
state:=2;
END_IF;
2: // Select on/off
voiceTalk(message:="SelectOnOff.wav");
key :=dtmfGetKey(timeout:=7000);
IF key=0 OR key=1 THEN
lamp:=BOOL(key);
state:=3;
ELSIF key <> -1 THEN
voiceTalk(message:="Illegal.wav");
END_IF;
3: // Command done
voiceTalk(message:="AllDone.wav");
gsmHangup();
state:=1;
END_CASE;
// if the user has terminated the connection we force the state back to
// the inital state (waiting for call)
IF NOT gsmOffHook() THEN
state:=1;
END_IF;
END_FUNCTION;
//---------------------------------------------------------------------------
// Detect intrusion in the greenhouse, and send an SMS message
//---------------------------------------------------------------------------
FUNCTION BurglerAlarm;
VAR
j : INT;
END_VAR;
// If burglaralarm is activated and we have an alarm:
IF Alarm_keyswitch AND Doorcontact THEN
// We will try 3 times to deliver the alarm:
FOR j:=1 TO 3 DO
// If call is being answered
IF gsmMakeCall(phonenumber:=PhoneNo) THEN
// Deliver the message
voiceTalk(message:="BurglerDetected.wav");
gsmHangup();
EXIT;
END_IF;
END_FOR;
END_IF;
END_FUNCTION;
//---------------------------------------------------------------------------
// The main program. This just calls the various functions sequentially.
//---------------------------------------------------------------------------
PROGRAM greenhouse2;
// Initialization code:
// Set the timer
// (Convert to seconds)
timer(pt := Alarm_time*60*1000);
// turn on GSM-module
gsmPower(power := ON);
BEGIN
timer();
sms();
voicecall();
OffHook := gsmOffHook();
Connected := gsmConnected();
// Control the temperature
TemperatureControl();
// Control lamp using SMS messages
SMSLamp();
// Control lamp using Voice-response system
VoiceLamp();
// Detect burgler alarm
BurglerAlarm();
END;
END_PROGRAM;
|
|