This function shows a dialog that shows a keyboard for the user to enter a text string.
The dialog closes either when the user selects OK or Cancel, or when it times out.
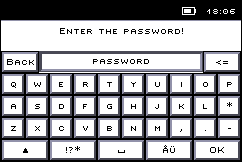
The buttons on the bottom of the keyboard besides the OK and space(␣) buttons are used to switch between different layouts:
▲
|
Click to switch between lower and upper case letters. For the symbol layout, it switches between two different sets of symbols.
|
!?*
|
Click to switch between the normal layout and the symbol layout. The symbol layout contains the numbers as well as common punctuation. The second page (accessed by pressing ▲) contains currency symbols and additional symbols.
|
ÅÜ
|
Click to switch between the normal layout and the language specific letters and diacritics. To select the language to use, keep the button pressed for about 3 seconds. This shows a list of the available language groups. The selection made in this list is remembered across resets. ▲ switches between upper and lower case letters.
|
Input:
message : STRING
The message to show to the user
timeout : INT (-1, 0..3600) default -1
The number of seconds without activity before the dialog closes by itself. Use -1 for no timeout.
max_len : INT (-1..254)
The maximum length of the string. Use -1 to get the maximum length.
text : STRING
The initial text to show.
Output:
text : STRING
The new text.
Returns: INT
2
|
- The Back button was clicked.
|
1
|
- The OK button was clicked.
|
0
|
- Timeout.
|
-1
|
- Interface is not open (see guiOpen).
|
-3
|
- Invalid timeout or max_len is invalid.
|
-8
|
- The text contains invalid characters.
|
-9
|
- A dialog is already being shown.
|
-11
|
- The GUI API is not supported.
|
Declaration:
FUNCTION guiShowTextInput : INT;
VAR_INPUT
message : STRING;
timeout : INT := -1;
max_len : INT := -1;
text : ACCESS STRING;
END_VAR;
Example:
INCLUDE rtcu.inc
PROGRAM test;
VAR
rc : INT;
text : STRING;
END_VAR;
BEGIN
...
text := "";
rc := guiShowTextInput(message := "Enter the password!", timeout:=60,
text := text, max_len := 10);
IF rc = 1 THEN
IF text = "password" THEN
DebugMsg(message:="Password was correct");
ELSE
DebugMsg(message:="Password was not correct");
END_IF;
END_IF;
...
END;
END_PROGRAM;
|